Learn C++ Programming – An Intermediate Journey
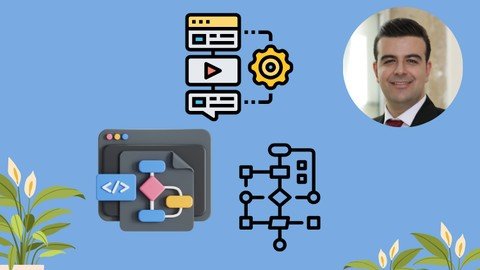
Free Download Learn C++ Programming – An Intermediate Journey
Published: 12/2024
MP4 | Video: h264, 1920x1080 | Audio: AAC, 44.1 KHz
Language: English | Size: 3.29 GB | Duration: 7h 24m
Bridge the gap to advanced C++: A comprehensive guide to intermediate C++ programming
What you'll learn
Understand the fundamentals and applications of one-dimensional and two-dimensional arrays in C++.
Learn to perform operations such as linear search, bubble sort, and calculating statistical measures using arrays.
Master the use of pointers to manipulate arrays, strings, and functions in C++.
Understand dynamic memory allocation and manage memory effectively in C++ programs.
Gain proficiency in working with strings, including string manipulation and file handling techniques.
Develop a solid understanding of enumerated types, structures, and unions to manage complex data.
Learn how to pass arrays, pointers, and structures as function parameters.
Implement advanced concepts like multi-dimensional arrays and nested structures in C++ applications.
Requirements
Basic knowledge of C++ is required to take this course.
Learners should be familiar with: Data types and basic operations
Learners should be familiar with: Control structures such as if-else and switch conditions
Learners should be familiar with: Looping constructs like for, while, and do-while loops
Learners should be familiar with: Functions, including their definition and use
Description
Are you ready to take your C++ skills to the next level? This course is designed for intermediate learners who have a solid understanding of the basics of C++ and want to bridge the gap to advanced-level programming. With a structured curriculum and hands-on examples, this course will empower you to write more efficient, scalable, and robust programs.
What you'll learn
in This CourseThis course focuses on mastering the key intermediate concepts of C++, including:Arrays: Explore one-dimensional, two-dimensional, and multi-dimensional arrays with real-world applications.Pointers: Learn how to manipulate memory efficiently, pass pointers to functions, and work with pointers in arrays and structures.Dynamic Memory: Understand how to allocate and manage memory effectively using advanced techniques.Strings: Perform string manipulation and use file handling to manage data in real-world scenarios.Vectors: Dive into complex data management with vectors.Highlights of the CourseReal-world examples: Apply concepts like sorting, searching, and statistical calculations using arrays.Practical exercises: Work on a large number of practical coding exercises to improve your coding skills. Comprehensive curriculum: Understand the interplay of arrays, pointers, and structures in developing robust C++ programs.Step-by-step guidance: Build confidence with complex topics through clear explanations and hands-on coding.Why Enroll in This Course?Tailored for intermediate learners: Designed to solidify foundational concepts and prepare you for advanced-level programming.Hands-on approach: Gain practical coding experience by implementing complex concepts step by step right after its corresponding lecture.Bridge to advanced C++: This course serves as a pathway to mastering topics like object-oriented programming and advanced algorithms.Who Should Take This Course?This course is perfect for:Intermediate C++ learners who want to advance their programming skills.Students preparing for advanced C++ courses or real-world programming challenges.Aspiring developers seeking to understand C++ memory management, advanced structures, and efficient coding techniques.Hobbyists or professionals eager to refine their problem-solving skills and take on complex programming challenges.PrerequisitesTo get the most out of this course, learners should already be familiar with:Basic C++ programming, including data types, if-else conditions, loops, switch statements, and functions.Embark on your C++ programming journey and equip yourself with the skills to solve complex problems, build scalable applications, and prepare for advanced programming concepts. Enroll today and take a step closer to becoming a C++ expert!Overview
Section 1: Control structure and iteration
Lecture 1 Use of `continue'
Lecture 2 Use of `break'
Lecture 3 Use of `goto'
Section 2: Arrays
Lecture 4 One dimensional array
Lecture 5 Coding exercise #1
Lecture 6 Coding exercise #2
Lecture 7 Array and function
Lecture 8 Coding exercise #3
Lecture 9 Coding exercise #4
Lecture 10 Coding exercise #5
Lecture 11 array and statics
Lecture 12 Coding exercise #6
Lecture 13 Coding exercise #7
Lecture 14 Coding exercise #8: Array manipulation techniques: part 1 (sorting)
Lecture 15 Coding exercise #9: Array manipulation techniques: part 2 (sorting)
Lecture 16 Coding exercise #10: Array manipulation techniques: part 3 (sorting)
Lecture 17 Coding exercise #11: Array manipulation techniques: part 4 (reversing)
Lecture 18 Coding exercise #12: Inserting an array in the beginning of another array
Lecture 19 Coding exercise #13: Inserting an array at the end of another array
Lecture 20 Coding exercise #14: Inserting an array in the middle of another array
Lecture 21 Coding exercise #15: Constant arrays
Lecture 22 Coding exercise #16: Two dimensional arrays
Lecture 23 Coding exercise #17: Coding exercise of two dimensional arrays
Lecture 24 Coding exercise #18: two-dimensional arrays exercise
Lecture 25 Coding exercise #19: Multi-dimensional arrays beyond two dimenstions
Section 3: Pointers
Lecture 26 Pointers
Lecture 27 Coding exercise #20: Pointers and functions
Lecture 28 Coding exercise #21: Pointers and functions
Lecture 29 Coding exercise #22: Pointers and functions
Lecture 30 Coding exercise #23: pointers and arrays
Lecture 31 Coding exercise #24: pointers and two-dimensional arrays
Lecture 32 Coding exercise #25: pointers and two dimensional arrays
Lecture 33 Coding exercise #25: Array of function pointers
Lecture 34 Coding exercise #26: Array of function pointers
Lecture 35 Coding exercise #27: Pointers to a function as a parameter of another function
Lecture 36 Coding exercise #28: Pointers to a function as a parameter of another function
Lecture 37 Coding exercise #29: Dynamic memory allocation using a pointer for arrays
Lecture 38 Coding exercise #30: Dynamic memory allocation using a pointer for arrays
Lecture 39 Coding exercise #31: A pointer to another pointer
Lecture 40 Coding exercise #32: A pointer to another pointer
Lecture 41 Coding exercise #33: Type casting
Lecture 42 Coding exercise #34: Type casting for void pointers
Lecture 43 Coding exercise #35: Type casting for pointers
Lecture 44 Coding exercise #35: Type casting for pointers
Lecture 45 Coding exercise #36: Type casting for pointers
Section 4: Strings
Lecture 46 Strings
Lecture 47 Coding exercise #37: String handling functions - length
Lecture 48 Coding exercise #38: String handling functions - access characters
Lecture 49 Coding exercise #39: String handling functions - append
Lecture 50 Coding exercise #40: String handling functions - insert
Lecture 51 Coding exercise #41: String handling functions - erase
Lecture 52 Coding exercise #42: String handling functions - replace
Lecture 53 Coding exercise #43: String handling functions - substring
Lecture 54 Coding exercise #44: String handling functions - clearing
Lecture 55 Coding exercise #45: String handling functions - reversing
Lecture 56 Coding exercise #46: String handling functions - sorting
Lecture 57 Coding exercise #47: An array of strings
Lecture 58 Coding exercise #48: An array of strings
Lecture 59 Coding exercise #49: An array of strings
Section 5: Vectors
Lecture 60 Coding exercise #50: push_back values to a vector
Lecture 61 Vectors
Lecture 62 Coding exercise #51: Accessing elements of a vector
Lecture 63 Coding exercise #52: Measure the size of a vector
Lecture 64 Coding exercise #53: Modify elements of a vector
Lecture 65 Coding exercise #54: Pop_back in a vector
Lecture 66 Coding exercise #55: Clearing a vector
Lecture 67 Coding exercise #56
Lecture 68 Coding exercise #57
Lecture 69 Coding exercise #58
Intermediate learners with a basic understanding of C++ programming.,Students aiming to strengthen their skills and transition to advanced C++ concepts.,Aspiring software developers seeking practical knowledge of intermediate C++ applications.,Programmers looking to bridge foundational topics and advanced-level programming.,Hobbyists or professionals eager to enhance their problem-solving skills in C++.
Homepage:
https://www.udemy.com/course/learn-c-programming-an-intermediate-journey/
DOWNLOAD NOW: Learn C++ Programming – An Intermediate Journey
Rapidgator
pcsjt.Learn.C.Programming..An.Intermediate.Journey.part2.rar.html
pcsjt.Learn.C.Programming..An.Intermediate.Journey.part4.rar.html
pcsjt.Learn.C.Programming..An.Intermediate.Journey.part3.rar.html
pcsjt.Learn.C.Programming..An.Intermediate.Journey.part1.rar.html
Fikper
pcsjt.Learn.C.Programming..An.Intermediate.Journey.part4.rar.html
pcsjt.Learn.C.Programming..An.Intermediate.Journey.part2.rar.html
pcsjt.Learn.C.Programming..An.Intermediate.Journey.part3.rar.html
pcsjt.Learn.C.Programming..An.Intermediate.Journey.part1.rar.html
Learn C++ Programming – An Intermediate Journey Torrent Download , Learn C++ Programming – An Intermediate Journey Watch Free Online , Learn C++ Programming – An Intermediate Journey Download Online
In today's era of digital learning, access to high-quality educational resources has become more accessible than ever, with a plethora of platforms offering free download video courses in various disciplines. One of the most sought-after categories among learners is the skillshar free video editing course, which provides aspiring creators with the tools and techniques needed to master the art of video production. These courses cover everything from basic editing principles to advanced techniques, empowering individuals to unleash their creativity and produce professional-quality content.
Comments (0)
Users of Guests are not allowed to comment this publication.